Applets
Definition of Applet
An applet is a small Java program that is a part of a web page and is downloaded automatically from a server when the client requests that web page.
Applet Fundamentals
Since applet is a part of a webpage, we will learn basic things related to web pages. A web page is a collection of information (ex: Google home page, Yahoo home page etc). Web pages are generally created using HTML.
A web page contains at least four basic HTML tags:
- <html> : Specifies that it is the root tag.
- <head> : Specifies the head section of the web page. It contains meta information, page title, scripts, external style sheet links etc.
- <body> : Contains the content that is displayed to a user who is visiting the web page.
- <title> : Specifies the title of the web page. It is specified inside <head> tags.
As an example let’s look at the HTML code of a web page that displays “Hello World” in large letters. The code is as follows:
hello.html
The file will be saved with .html extension. When you open the file with a web browser, the output will be as follows:
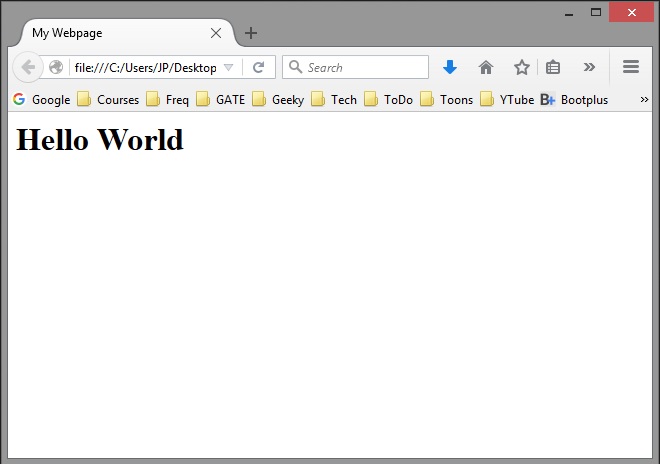
An applet can be displayed in a web page using the <applet> tag as shown below:
<applet code=”ClassName” height=”200″ width=”400″></applet>
An applet doesn’t contain a main() method like the Java console programs. An applet is used to display graphics or to display a GUI (ex: login form, registration form) to the users.
Creating an Applet
An applet can be created by extending either Applet class or JApplet class. First let’s see how to create an applet using the Applet class. The Applet class is available in java.applet package. An applet which displays “Hello World” is shown below:
MyApplet.java
Remember to include the <applet> tag in comments. This is useful for running the applet. The value of code attribute must match with the class name.
In the above applet program, the class MyApplet extends the Applet class and it contains a method named paint() which accepts a parameter of the type Graphics. The Graphics class belongs to java.awt package and is used to display text or graphics on our applet.
The paint() method is used to re-display the output when the applet is resized or minimized or maximized. The drawString() method belongs to Graphics class and it displays “Hello World!” on the applet display area at the point whose coordinates are (20, 20).
Save the file as MyApplet.java and compile it to generate MyApplet.class file.
Running an Applet
An applet can be executed in two ways:
- Using appletviewer command-line tool or
- Using a browser
Using appletviewer tool:
Java provides a command line tool named appletviewer for quick debugging of applets. Use the following syntax for running an applet using appletviewer:
appletviewer filename
As the file name of our applet is MyApplet.java, the command for executing the applet is:
appletviewer MyApplet.java
Output of the above command will be as shown below:
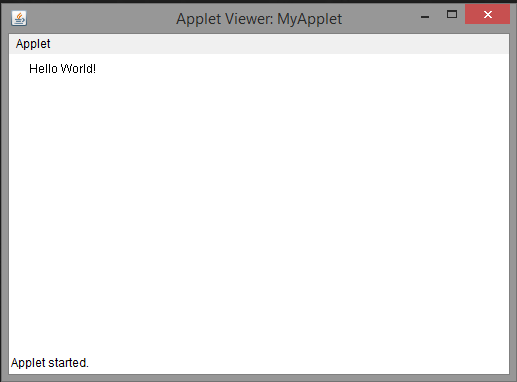
Using a browser:
Create a web page named hello.html with the following HTML code:
Unfortunately from Java 7 onwards, local applets (unsigned) cannot be viewed using a browser and Google Chrome browser no longer supports applets in web pages.
It is recommended to use appletviewer tool to debug and execute applets.
Differences between a Java Application and Applets
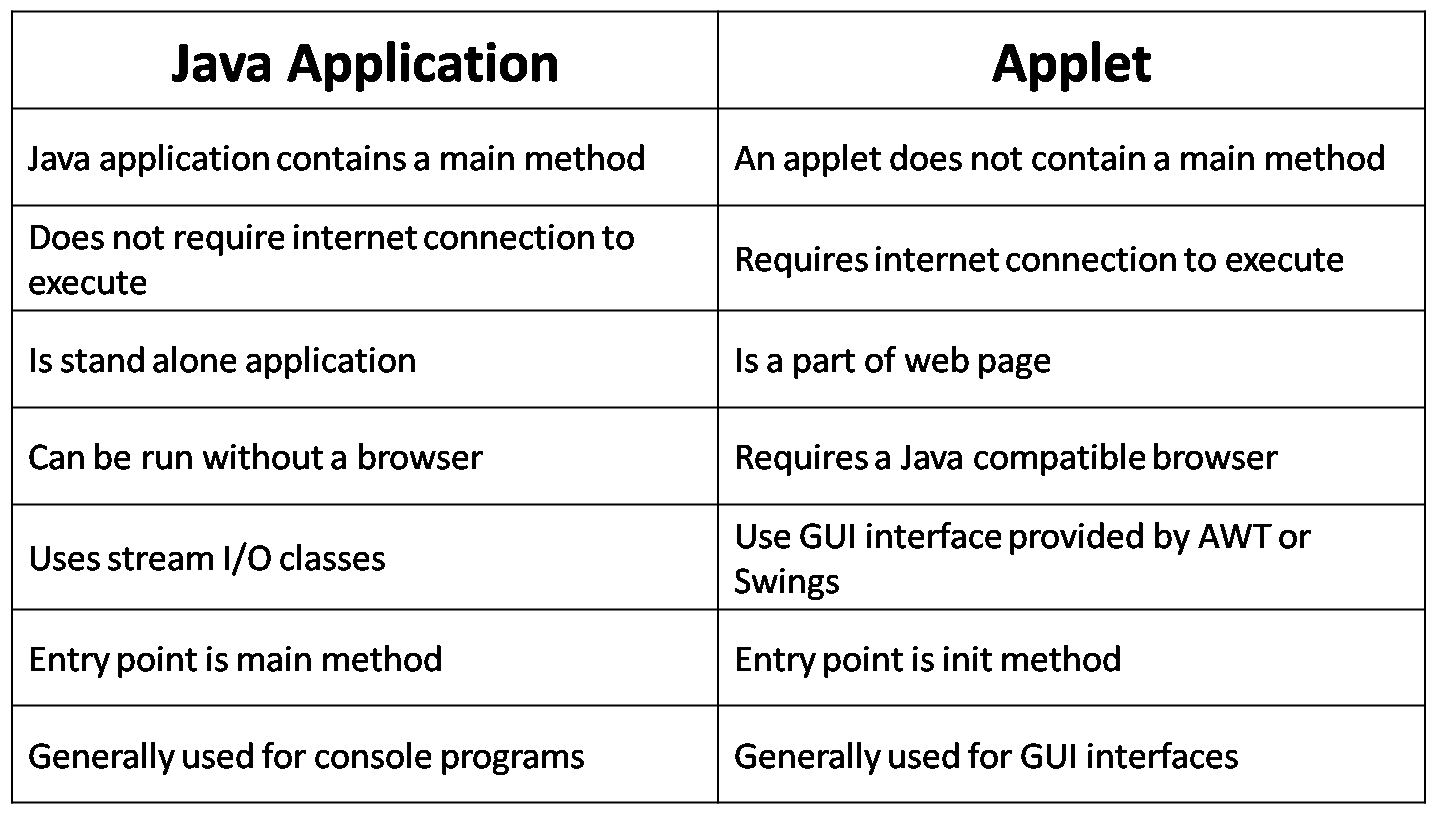
Applet Class
The Applet class provides the support for creation and execution of applets. It allows us to start and stop the execution of an applet. Applet class extends the Panel class which extends Container class and which in turn extends the Component class. So applet supports all window-based activities.
Methods in Applet Class
Below are some of the methods available in the class Applet:
- init() – This method is called when the execution of the applet begins. It is the entry point for all applets.
- start() – This method is automatically executed after the init() method completes its execution or whenever the execution of an applet is resumed.
- stop() – This method executes whenever the execution of the applet is suspended.
- destroy() – This method is called when the webpage containing the applet is closed.
- getCodeBase() – Returns the URL associated with the applet.
- getDocumentBase() – Returns the URL of the HTML document that invokes the applet.
- getParameter(String paramName) – Returns the value associated with the paramName. Returns null if there is no value.
- isActive() – Returns true if the applet is started or false if the applet is stopped.
- resize(int width, int height) – Resizes the applet based on the given width and height.
- showStatus(String str) – Displays the string str in the status bar of the browser or applet.
Passing Parameters to Applets
Parameters specify extra information that can be passed to an applet from the HTML page. Parameters are specified using the HTML’s param tag.
Param Tag
The <param> tag is a sub tag of the <applet> tag. The <param> tag contains two attributes: name and value which are used to specify the name of the parameter and the value of the parameter respectively. For example, the param tags for passing name and age parameters looks as shown below:
<param name=”name” value=”Ramesh” />
<param name=”age” value=”25″ />
Now, these two parameters can be accessed in the applet program using the getParameter() method of the Applet class.
getParameter() Method
The getParameter() method of the Applet class can be used to retrieve the parameters passed from the HTML page. The syntax of getParameter() method is as follows:
String getParameter(String param-name)
Let’s look at a sample program which demonstrates the <param> HTML tag and the getParameter() method:
Output of the above program is as follows:
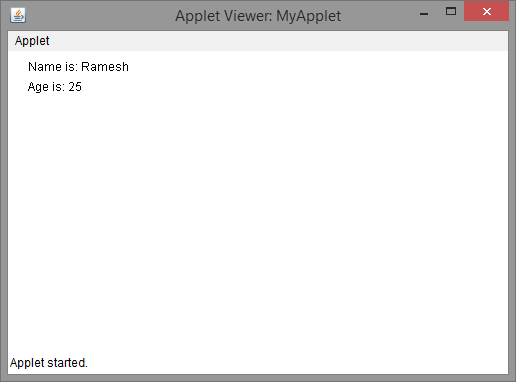
Applet Tag
applet Tag Attributes
Following are the various attributes of applet tag:
- code: Used to specify the name of the applet class.
- codebase: Used to specify the location at which the applet code (class) is available.
- width: Used to specify the width of the applet display area.
- height: Used to specify the height of the applet display area.
- name: Used to specify the name of the applet which is helpful in distinguish one applet from another when there are multiple applets in the same webpage.
- hspace: Used to specify the horizontal space to the left and right of the applet display area.
- vspace: Used to specify the vertical space to the top and bottom of the applet display area.
- align: Used to specify the alignment of the applet display area with respect to other HTML elements.
Above listed attributes are the most frequently used attributes of the applet tag. Below is sample HTML code using some of the attributes specified above.
<applet code=”MyApplet” codebase=”http://www.abc.com/applets/”height=”300″ width=”400″name=”applet1″hspace=”20″ vspace=”20″></applet>
Comments
Post a Comment